mirror of
https://git.ryujinx.app/ryubing/ryujinx.git
synced 2025-05-02 14:07:42 +02:00
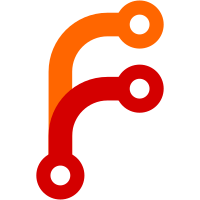
* Implement NGC service * Use raw byte arrays instead of string for _wordSeparators * Silence IDE0230 for _wordSeparators * Try to silence warning about _rangeValuesCount not being read on SparseSet * Make AcType enum private * Add abstract methods and one TODO that I forgot * PR feedback * More PR feedback * More PR feedback
54 lines
1.3 KiB
C#
54 lines
1.3 KiB
C#
namespace Ryujinx.Horizon.Sdk.Ngc.Detail
|
|
{
|
|
class Bp
|
|
{
|
|
private readonly BpNode _firstNode = new();
|
|
private readonly SbvSelect _sbvSelect = new();
|
|
|
|
public bool Import(ref BinaryReader reader)
|
|
{
|
|
return _firstNode.Import(ref reader) && _sbvSelect.Import(ref reader);
|
|
}
|
|
|
|
public int ToPos(int index)
|
|
{
|
|
return _sbvSelect.Select(_firstNode.Set, index);
|
|
}
|
|
|
|
public int Enclose(int index)
|
|
{
|
|
if ((uint)index < (uint)_firstNode.Set.BitVector.BitLength)
|
|
{
|
|
if (!_firstNode.Set.Has(index))
|
|
{
|
|
index = _firstNode.FindOpen(index);
|
|
}
|
|
|
|
if (index > 0)
|
|
{
|
|
return _firstNode.Enclose(index);
|
|
}
|
|
}
|
|
|
|
return -1;
|
|
}
|
|
|
|
public int ToNodeId(int index)
|
|
{
|
|
if ((uint)index < (uint)_firstNode.Set.BitVector.BitLength)
|
|
{
|
|
if (!_firstNode.Set.Has(index))
|
|
{
|
|
index = _firstNode.FindOpen(index);
|
|
}
|
|
|
|
if (index >= 0)
|
|
{
|
|
return _firstNode.Set.Rank1(index) - 1;
|
|
}
|
|
}
|
|
|
|
return -1;
|
|
}
|
|
}
|
|
}
|