mirror of
https://git.ryujinx.app/ryubing/ryujinx.git
synced 2025-07-15 06:06:28 +02:00
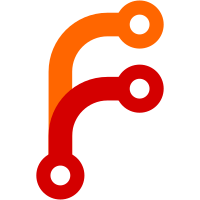
* misc: Move Ryujinx project to Ryujinx.Gtk3 This breaks release CI for now but that's fine. Signed-off-by: Mary Guillemard <mary@mary.zone> * misc: Move Ryujinx.Ava project to Ryujinx This breaks CI for now, but it's fine. Signed-off-by: Mary Guillemard <mary@mary.zone> * infra: Make Avalonia the default UI Should fix CI after the previous changes. GTK3 isn't build by the release job anymore, only by PR CI. This also ensure that the test-ava update package is still generated to allow update from the old testing channel. Signed-off-by: Mary Guillemard <mary@mary.zone> * Fix missing copy in create_app_bundle.sh Signed-off-by: Mary Guillemard <mary@mary.zone> * Fix syntax error Signed-off-by: Mary Guillemard <mary@mary.zone> --------- Signed-off-by: Mary Guillemard <mary@mary.zone>
51 lines
1.7 KiB
C#
51 lines
1.7 KiB
C#
using Avalonia.Controls;
|
|
using Avalonia.Input;
|
|
using Avalonia.Interactivity;
|
|
using Ryujinx.Ava.UI.Helpers;
|
|
using Ryujinx.Ava.UI.ViewModels;
|
|
using Ryujinx.UI.App.Common;
|
|
using System;
|
|
|
|
namespace Ryujinx.Ava.UI.Controls
|
|
{
|
|
public partial class ApplicationGridView : UserControl
|
|
{
|
|
public static readonly RoutedEvent<ApplicationOpenedEventArgs> ApplicationOpenedEvent =
|
|
RoutedEvent.Register<ApplicationGridView, ApplicationOpenedEventArgs>(nameof(ApplicationOpened), RoutingStrategies.Bubble);
|
|
|
|
public event EventHandler<ApplicationOpenedEventArgs> ApplicationOpened
|
|
{
|
|
add { AddHandler(ApplicationOpenedEvent, value); }
|
|
remove { RemoveHandler(ApplicationOpenedEvent, value); }
|
|
}
|
|
|
|
public ApplicationGridView()
|
|
{
|
|
InitializeComponent();
|
|
}
|
|
|
|
public void GameList_DoubleTapped(object sender, TappedEventArgs args)
|
|
{
|
|
if (sender is ListBox listBox)
|
|
{
|
|
if (listBox.SelectedItem is ApplicationData selected)
|
|
{
|
|
RaiseEvent(new ApplicationOpenedEventArgs(selected, ApplicationOpenedEvent));
|
|
}
|
|
}
|
|
}
|
|
|
|
public void GameList_SelectionChanged(object sender, SelectionChangedEventArgs args)
|
|
{
|
|
if (sender is ListBox listBox)
|
|
{
|
|
(DataContext as MainWindowViewModel).GridSelectedApplication = listBox.SelectedItem as ApplicationData;
|
|
}
|
|
}
|
|
|
|
private void SearchBox_OnKeyUp(object sender, KeyEventArgs args)
|
|
{
|
|
(DataContext as MainWindowViewModel).SearchText = (sender as TextBox).Text;
|
|
}
|
|
}
|
|
}
|